Using videos in a Silverlight based application is a very exciting feature. In this article, we will learn how we can control the playback of movie using some coding in code-behind. Let's see how!
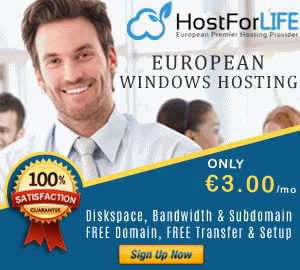
Dealing with Automatic Start
By default videos automaticall get started when we run the project, it is distracting feature from the users point of view. To change auto start false, select the video on stage and in properties uncheck the 'AutoPlay' option.
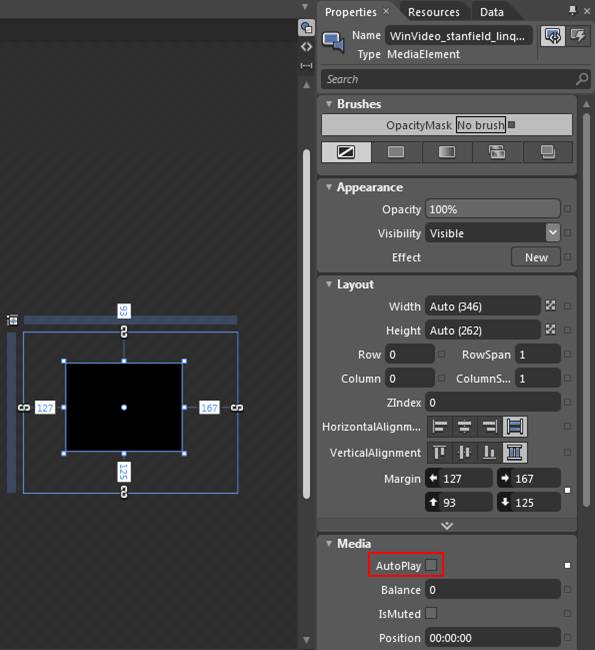
Dealing with Endless Playback
By default, when the video reaches the end then it stops and does not start again. To change such a setting follow the steps:
1. Select the video on stage
2. In Properties, switch the mode from 'Properties' to 'Events'.
3. In the Event list, point to 'MediaEnded' label and type the event handler name (I will be using md_ended_eve) and then press tab to apply it and it automatically switches you to code-behind with a new event.
4. Now type the following code inside event handler:
(intro_wmv).Stop();
(intro_wmv).Play();
In above code 'intro_wmv' is my media fine name.
5. Now test the application.
XAML Code
<Grid
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="SilverlightApplication1.MainPage"
Width="640" Height="480">
<MediaElement x:Name="intro_wmv"
Margin="54,64,104,60"
Source="/intro.wmv"
Stretch="Fill"
MediaEnded="md_ended_eve" AutoPlay="False"/>
</Grid>
XAML.CS Code
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SilverlightApplication1
{
public partial class MainPage : Grid
{
public MainPage()
{
// Required to initialize variables
InitializeComponent();
}
private void md_ended_eve(object sender, System.Windows.RoutedEventArgs e)
{
// TODO: Add event handler implementation here.
(intro_wmv).Stop();
(intro_wmv).Play();
}
}
}
Control Video Playback by Pause and Play
By default in Silverlight video plays and we can't control it by pausing and playing. But by writing some lines in code-behind we can control this playback. For this we have to create the event as we saw in above example. Let's follow the steps:
1. Open the event explorer by switching the property (look above image).
2. Type the event name in 'MouseLeftButtonDown', I will be using here 'pause_start_evn' and press tab to switch in event handler mode.
3. In the appeared event type the following code:
private bool IsPaused=true;
private void pause_start_evn(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
// TODO: Add event handler implementation here.
if(IsPaused)
{
(intro_wmv as MediaElement).Play();
IsPaused=false;
}
else
{
(intro_wmv as MediaElement).Pause();
IsPaused=true;
}
}
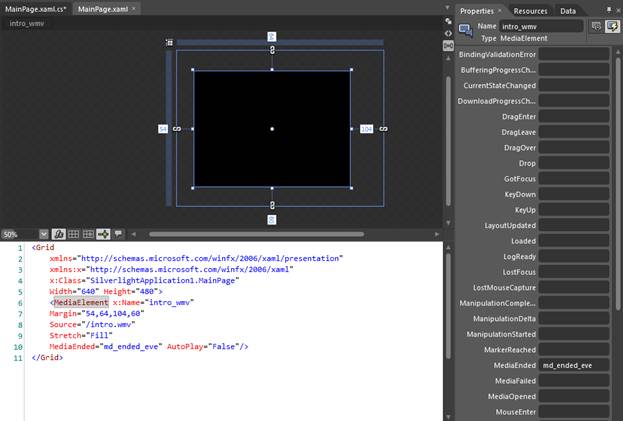
4. Now test the application and check by right mouse click on video surface.
XAML Code
<Grid
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="SilverlightApplication1.MainPage"
Width="640" Height="480">
<MediaElement x:Name="intro_wmv"
Margin="54,64,104,60"
Source="/intro.wmv"
Stretch="Fill"
MediaEnded="md_ended_eve"
MouseLeftButtonDown="pause_start_evn"/>
</Grid>
XAXM.CS Code
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SilverlightApplication1
{
public partial class MainPage : Grid
{
public MainPage()
{
// Required to initialize variables
InitializeComponent();
}
private void md_ended_eve(object sender, System.Windows.RoutedEventArgs e)
{
// TODO: Add event handler implementation here.
(intro_wmv).Stop();
(intro_wmv).Play();
}
private bool IsPaused=true;
private void pause_start_evn(object sender, System.Windows.Input.MouseButtonEventArgs e)
{
// TODO: Add event handler implementation here.
if(IsPaused)
{
(intro_wmv as MediaElement).Play();
IsPaused=false;
}
else
{
(intro_wmv as MediaElement).Pause();
IsPaused=true;
}
}
}
}
Now, that's all about the controlling of video playback in a Silverlight based application. Happy coding!
HostForLIFE.eu Silverlight 6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
