
October 18, 2019 11:42 by
Peter
We can integrate media into our Silverlight pages and WPF UserControls. The MediaElement object provides several media-specific properties. The following list describes the commonly used properties.
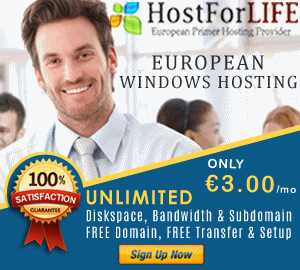
- AutoPlay: Specifies whether the MediaElement should begin playing automatically. The default value is True.
- IsMuted: Specifies whether the MediaElement is silenced. A value of True mutes the MediaElement. The default value is False.
- Stretch: Specifies how video is stretched to fill the MediaElement object. Possible values are None, Uniform, UniformToFill, and Fill. The default is Fill.
- Volume: Specifies the volume of the MediaElement object’s audio as a value from 0 to 1, with 1 being the loudest. The default value is 0.5.
In addition to its media-specific properties, MediaElement also has all the properties of a UIElement, such as Opacity and Clip.
Controlling Media Playback
You can control media playback by using the Play, Pause, and Stop methods of a MediaElement object.
<Canvas
xmlns="http://schemas.microsoft.com/client/2007"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Loaded="CanvasLoaded">
<MediaElement x:Name="MyMedia" Stretch="Uniform"
Source="/images/Silverlight_Small.wmv" Height="200" Width="200" />
<Canvas x:Name="ButtonPanel">
<Canvas Background="Red" Canvas.Left="10" Canvas.Top="185" Height="25" Width="50"
MouseLeftButtonDown="StopMedia" Cursor="Hand">
<Rectangle Height="30" Width="40" Canvas.Left="10" />
<TextBlock Text="Stop" Canvas.Left="10" Foreground="Yellow" />
</Canvas>
<Canvas Background="Green" Canvas.Left="70" Canvas.Top="185" Height="25" Width="50"
MouseLeftButtonDown="PlayMedia" Cursor="Hand">
<Rectangle Height="30" Width="40" Canvas.Left="10" />
<TextBlock Text="Play" Canvas.Left="10" Foreground="Yellow" />
</Canvas>
<Canvas Background="Blue" Canvas.Left="130" Canvas.Top="185" Height="25" Width="60"
MouseLeftButtonDown="PauseMedia" Cursor="Hand">
<Rectangle Height="30" Width="40" Canvas.Left="10" />
<TextBlock Text="Pause" Canvas.Left="10" Foreground="Yellow" />
</Canvas>
<Canvas Background="Black" Canvas.Left="10" Canvas.Top="215" Height="25" Width="180"
MouseLeftButtonDown="ToggleFullScreen" Cursor="Hand">
<Rectangle Height="30" Width="40" Canvas.Left="10" />
<TextBlock Text="Full Screen" Canvas.Left="55" Foreground="Yellow" />
</Canvas>
</Canvas>
</Canvas>
// --------- JavaScript Code to control the Media object -------------
// Fires when Canvas is loaded
function CanvasLoaded(sender, args)
{
var canvas = sender.getHost();
canvas.content.onfullScreenChange = onFullScreenChanged;
}
// Fires when Full screen is changed
function onFullScreenChanged(sender, args)
{
var canvas = sender.getHost();
var buttonPanel = sender.findName("ButtonPanel");
// Change the opacity of the button panel so that in the full screen it disappears and in normal screen it appears
if (canvas.content.fullScreen == true)
buttonPanel.opacity = 0;
else
buttonPanel.opacity = 1;
// change the media object height and width to the canvas height and width
var media = sender.findName("MyMedia");
media.width = canvas.content.actualWidth;
media.height = canvas.content.actualHeight;
}
//Fires when Full Screen button is clicked
function ToggleFullScreen(sender, args)
{
var canvas = sender.getHost();
canvas.content.fullScreen = !canvas.content.fullScreen;
}
// Fires when Stop button is clicked
function StopMedia(sender, args)
{
sender.findName("MyMedia").stop();
}
// Fires when Play button is clicked
function PlayMedia(sender, args)
{
sender.findName("MyMedia").play();
}
// Fires when Pause button is clicked
function PauseMedia(sender, args)
{
sender.findName("MyMedia").pause();
Hope this helps!!