Do you want to make a game using Silverlight? At the first, you need something that moves around the screen. For example, you need at least four buttons that move a sprite in four directions. How do you do that? Well, there are multiple ways to accomplish this movement, some more flexible than others. Today, I’m going to tell you how to use Silverlight animations to do that job.
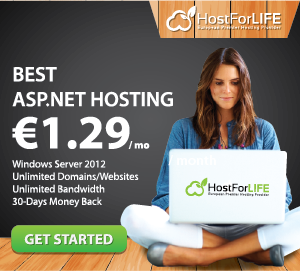
Silverlight animations are not hard to use, but what is hard to master are the dynamic animations, which require multiple classes to create and set up. You have to set up a storyboard, create an animation, and then set the target information. When it is all said and done, you will end up with something like this:
[silverlight width="400" height="300" src="aMovement1.xap" border="true"]
As with any Silverlight app, we need to start with some Xaml:
<Canvas Height="300" Name="canvas1" Width="400" >
<Canvas.Resources>
<Storyboard x:Name="mySB"></Storyboard>
</Canvas.Resources>
<Ellipse x:Name="myEllipse" Width="50" Height="50" Canvas.Left="175">
<Canvas.Top="125" Fill="Black" />
</Canvas>
I know what you are thinking, maybe this is not enough XAML for animations. Well, this is what we’re going to do. We are actually creating all of the animations dynamically and adding them to the storyboard. Then, to start with, we need an event (a keyboard triggered event).
What we need is a Key Up event, that only fires when we release a key, as opposed to pushing it down. Now I am using Visual Studio 2010 (which is currently under beta, so it is a free download), so I am not adding the event to the Canvas myself, but it is one line of code you can find. As I stated above, you need to setup a Key Up event. This event also has to be tied to the Canvas, so it works no matter where you click the application:
private void canvas1_KeyUp(object sender, KeyEventArgs e)
{
}
The first thing that we need to add to our event is key capturing. If you have ever done this before, you will recognize the code, if not it is extremely easy to do:
private void canvas1_KeyUp(object sender, KeyEventArgs e)
{
if (e.Key == Key.Left)
{
}
else if (e.Key == Key.Right)
{
}
else if (e.Key == Key.Up)
{
}
else if (e.Key == Key.Down)
{
}
}
What is going on here is that we are taking the key pressed, in this case a property of “e”, which is passed with the event. This gives up information about what key was pressed so we can compare it to key codes built into C#, which is given as an easy to use “e” num. As you can see, it is pretty obvious how to capture the right key.
Now we can start to setup some variables (movement speed and movement distance). Speed corresponds to how long it will take to travel a length equal to movement distance. For example we will set movement distance at 100 and speed at 0.5, making the animation travel 100 pixels in half a second. All we are doing right now is setting these variables, for later use:
private void canvas1_KeyUp(object sender, KeyEventArgs e)
{
Double mDist = 100.00;
Double mSpeed = 0.5;
if (e.Key == Key.Left)
{
}
else if (e.Key == Key.Right)
{
}
else if (e.Key == Key.Up)
{
}
else if (e.Key == Key.Down)
{
}
}
The next step is to start setting up the animation. To do this, we utilize a lot of different classes and methods like:
private void canvas1_KeyUp(object sender, KeyEventArgs e)
{
Double mDist = 100.00;
Double mSpeed = 0.5;
Double x = Canvas.GetLeft(myEllipse);
Double y = Canvas.GetTop(myEllipse);
DoubleAnimation animation = new DoubleAnimation();
animation.Duration = new Duration(TimeSpan.FromSeconds(mSpeed));
if (e.Key == Key.Left)
{
animation.From = x;
animation.To = x - mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.LeftProperty));
}
else if (e.Key == Key.Right)
{
animation.From = x;
animation.To = x + mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.LeftProperty))
}
else if (e.Key == Key.Up)
{
animation.From = y;
animation.To = y - mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.TopProperty));
}
else if (e.Key == Key.Down)
{
animation.From = y;
animation.To = y + mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.TopProperty));
}
Storyboard.SetTarget(animation, myEllipse);
mySB.Children.Add(animation);
mySB.Begin();
}
So starting from the top, the first thing we do is get the current position of our Ellipse. We can't really do very much without our object's current position. It is important to do this at a specific point, because the animation is of course going to change that position. Once we have the position, we begin to create the animation. Yes, there is a Double Animation object we can use, but that is not the only important object.
The first thing we do with our animation is set up its duration, which is done using the Duration object and Time span class. Using Time span's methods, we can set the duration to seconds, minutes, hours, or even days.
Now, it gets a little crazy when we get to the different movement directions. For each direction, we have to set the animation's To and From properties. Then we use some a static method in the Storyboard class called SetTargetProperty(), which allows us to tell the animation what property to animate on the target. For horizontal movement, that would be the LeftProperty, vertical the TopProperty. The tricky thing is that you have to use the Canvas class to get these properties. To make things even crazier, you have to pass it as an object called PropertyPath so you have to create that object as well. Then whole thing ends up being a web of objects and static methods.
Before we can finally add the animation to the storyboard, we have to set its target. In this case we are going to target our ellipse. We do this with the static method SetTargetin the Storyboard class. Once the target has been set, we add it to the storyboard, then start the animation.
If you ran the code we have now, you will notice one thing, it only works once. If you try to add the animation more than once, Silverlight doesn't really like it, so it fails. What we have to do is remove the current animation from the storyboard, or better yet, clear it entirely. This was the really tricky part.
In order to clear the storyboard, any animations attached have to be stopped. This is fine, but in order for things to work, we have to take the position of the ellipse before we clear the animations. So, we pause, take the position, then finally stop and clear the animations. But, one final step is setting the position of the ellipse. This has to do with animating only one axis at a time. While the animation is going, only one axis is truly updated, so we need to set the position to make sure we have the right coordinates for the animation. The final version will look something like so:
private void canvas1_KeyUp(object sender, KeyEventArgs e)
{
Double mDist = 100.00;
Double mSpeed = 0.5;
mySB.Pause();
Double x = Canvas.GetLeft(myEllipse);
Double y = Canvas.GetTop(myEllipse);
mySB.Stop();
mySB.Children.Clear();
Canvas.SetLeft(myEllipse, x);
Canvas.SetTop(myEllipse, y);
DoubleAnimation animation = new DoubleAnimation();
animation.Duration = new Duration(TimeSpan.FromSeconds(mSpeed));
if (e.Key == Key.Left)
{
animation.From = x;
animation.To = x - mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.LeftProperty));
}
else if (e.Key == Key.Right)
{
animation.From = x;
animation.To = x + mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.LeftProperty));
}
else if (e.Key == Key.Up)
{
animation.From = y;
animation.To = y - mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.TopProperty));
}
else if (e.Key == Key.Down)
{
animation.From = y;
animation.To = y + mDist;
Storyboard.SetTargetProperty(
animation, new PropertyPath(Canvas.TopProperty));
}
Storyboard.SetTarget(animation, myEllipse);
mySB.Children.Add(animation);
mySB.Begin();
}
This gives us the animated movement we are looking for. Not a lot of code, but there is a lot going on. After using 3 separate key classes, and even more objects, we can dynamically create and use animations to move our ellipse around the screen.
Easy right?
HostForLIFE.eu Silverlight 4 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
