Today, I will make a tutorial about how to make simple video player with Silverlight 4. Once you have that lets make the project, We want to make a Silverlight Application and after naming your project, on the next dialogue select Silverlight 4 from the combo box.
The Pre-created code for our project should look like this:

<UserControl x:Class="SilverlightApplication3.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/exp<b></b>ression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
</Grid>
</UserControl>
However, We don't need all those links, We only need a couple they are
view sourceprint?
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
So you can delete the others, Once you have your code should look like this:
view sourceprint?
<UserControl x:Class="SilverlightApplication3.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Grid x:Name="LayoutRoot" Background="White">
</Grid>
</UserControl>
After that minor tweak, We now need to add a Canvas tag to hold all of our video player elements together for us, To do this we need to put the <Canvas> Tag between the <Grid> Tags.
<Grid x:Name="LayoutRoot" Background="White">
<Canvas Name="Holder" Width="350" Height="220" Background="Black">
</Canvas>
</Grid>
These properties of the Canvas are very common in our project, All our elements will have a Name, A Height and a Width. They are measured in Pixels so you can easily make this pixel-perfect if you want to. The name is what we use to address the element of the project, We will need this later on. So We have in effect a black shape. We now need to add the video in, to do this we need to use the <MediaElement> tag. This have three main properties that we need in addition to a Name, Width and Height we need the Source, Volume and AutoPlay settings.
- The source is what we want the media element to find and play, In our case the video is at media/media.wmv so, Our source would look like this
Source="media/media.wmv"
- The volume setting is pretty self explanitoriy so we will set it to 100 for this tutorial.
- The AutoPlay setting has two values, True or False, It determines if the video should automatically play when it has loaded, for this tutorial we will set it to False.
So after all that, Our MediaElement Code should look like this
<MediaElement Name="Video" Source="media/media.wmv" AutoPlay="False" Volume="100" />
I have named the element video so that we will not get confused to it's function later on in the tutorial.
Right ok, Now we have a video lets add some controls so that we can control it. Using the resources I have provided, We will add a new image tag into our code, this will be our Play Button.
The code for this will be like so;
<Image Name="btnPlay" Height="17" Width="49" Source="media/play.png" />
As you can see, The element has a name, height, width and source. Because we have put all our resources into a folder in the project called media we always address the image as media/play.png instead of just play.png
We can also add other properties to this, As well as position it. To do so we will change the opacity to 0.4 and move it 220 pixels down from the canvas so we can see the button clearly. So, The code will now look like this;
<Image Name="btnPlay" Height="17" Width="49" Source="media/play.png" Opacity="0.4" Canvas.Top="220" />
The Opacity property can be any value between 0 and 1.
Right, We have an image that doesn't do anything which isn't entirely useful at the moment so lets add some code to it. Open the event window while the image is selected and find the event for MouseLeftButtonDown this is silverlights version of click. It will take you to the code behind the project for that event and this bit is very simple, the only code we need to put in this bit is Video.Play(); and thats all, That will make the video play! easy!
If your confused where to put it, this is what it should look like:
private void btnPlay_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
Video.Play();
}
You may notice that the source of the media element has an error on it, To fix this you find the media.wmv in the media folder and change it's Build Action to Resource and it will work fine.
You can run it now and the video will play.
HostForLIFE.eu Silverlight 4 Hosting
HostForLIFE.eu revolutionized hosting with Plesk Control Panel, a Web-based interface that provides customers with 24x7 access to their server and site configuration tools. Plesk completes requests in seconds. It is included free with each hosting account. Renowned for its comprehensive functionality - beyond other hosting control panels - and ease of use, Plesk Control Panel is available only to HostForLIFE's customers. They offer a highly redundant, carrier-class architecture, designed around the needs of shared hosting customers.
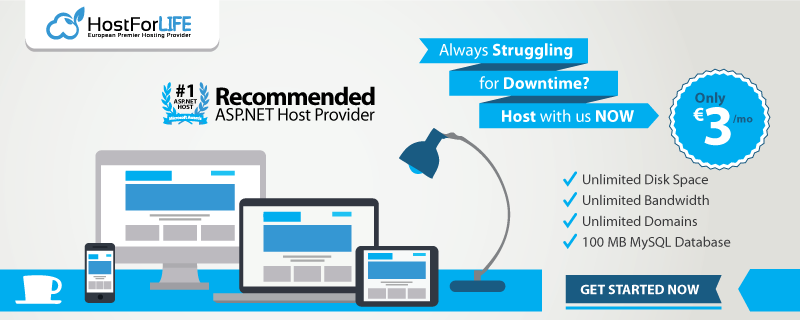