In Silverlight, due to security issues, an ordinary application is NOT allowed to write to/read from arbitrary locations on the file system (except through some dialogs). How do we save those data temporarily inside the file system (such as a text file) so that the application can retrieve and use it later? Sure you can use the Isolated Storage Feature in Silverlight.
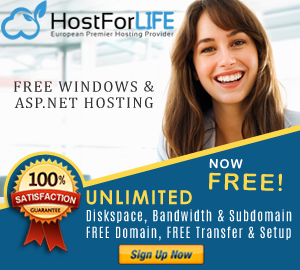
Isolated Storage allows you to access to a small segment of hard-disk space, which you will have no idea where the files are being stored. Usually you need it when you want to store small amounts of not essential information (eg: user-specific details, user preferences). Especially when you want to communicate with the web service, having the back-up storage can enable to page to reload the data without the user retyping again.
You can save DateTime into the memory and can retrieve it using Isolated Storage. Here is the example:'
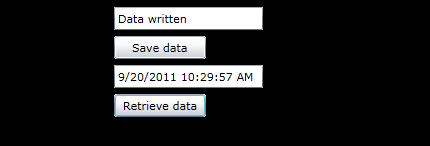
Step 1
First, you need to open an Isolated Store. You can use the IsolatedStorageFile class and call the static IsolatedStorageFile.GetUserStoreForApplication() method:
IsolatedStorageFile store = IsolatedStorageFile.GetUserStoreForApplication()
With this, you can use System.IO namespace to write and read data.
Step 2
Now, you have to write DateTime into memory as follows:
using (IsolatedStorageFile store = IsolatedStorageFile.GetUserStoreForApplication())
{
using (IsolatedStorageFileStream stream = store.CreateFile("Date.txt"))
{
StreamWriter writer = new StreamWriter(stream);
writer.Write(DateTime.Now);
writer.Close();
}
textBox1.Text = "Data written";
}
Step 3
Then, you can retrieve DateTime from memory, use this following code:
using (IsolatedStorageFile store = IsolatedStorageFile.GetUserStoreForApplication())
{
using (IsolatedStorageFileStream stream = store.OpenFile("Date.txt", FileMode.Open))
{
StreamReader reader = new StreamReader(stream);
textBox2.Text = reader.ReadLine();
reader.Close();
}
}
Simple right?
Silverlight 6 with Free ASP.NET Hosting
Try our Silverlight 6 with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
