There are many other great articles and blog posts on how to create simple Silverlight Splash Screens. This article adds on top of them and helps you design a more complex splash screen with Story Boards and Floating Text Blocks. I am not a great designer and thus I am taking a design que from Telerik's Silverlight Demos splash screen. They have some amazing designers and their splash screen is an amazing example of that. If you have not already noticed it please visit http://demos.telerik.com/silverlight/ and have a look yourself. We will try to replicate the same behavior in our splash screen. Here is a step-by-step guide to do this.
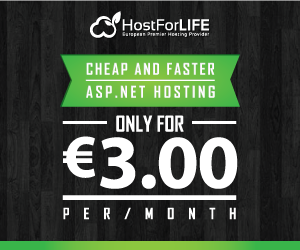
1. Create your XAML
In order to add the splash screen you will first need to add a new Silverlight 1.0 JavaScript (we will refer to it as SLJS for the sake of writing simplicity) page on your server side code.
Since you cannot modify this file in Blend, I created a new Silverlight project and created a new Silverlight User control in it.
Once I had completed the design of this page in Blend, I copied the content to the Silverlight JS page on the server side.
I had to make a few modifications that are listed below.
- First delete the x:class namespace as that is not supported on a SLJS page
- Also delete the underlying code file (in my case it was SplashScreen.xaml.cs)
- I added a storyboard animation to float the text from left to right
<Grid.Resources>
<Storyboardx:Name="sb1"RepeatBehavior="1x"Completed="onCompleted">
<DoubleAnimationDuration="0:0:5"To="255"
Storyboard.TargetProperty="X"Storyboard.TargetName="myTranslate"d:IsOptimized="True"/>
</Storyboard>
</Grid.Resources>
Based on your design, the following content may change (I designed it to match the same as Telerik's splash page):
<Grid Background="#FFF4F4F4" RenderTransformOrigin="0.5,0.5"> <Grid.RowDefinitions> <RowDefinition Height="120"/> <RowDefinition Height="32"/> <RowDefinition Height="8"/> <RowDefinition Height="20"/>
<RowDefinition Height="375*"/> </Grid.RowDefinitions> <TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Grid.Row="0" Text="Silverlight" VerticalAlignment="Top" Height="153" Width="806" FontSize="133.333"
Foreground="#FFEEEEEE" Grid.RowSpan="3"/> <!--<StackPanel Orientation="Horizontal" Grid.Row="1" Margin="100,0,0,0" >
<Image Source="/Content/np_logo.PNG" Margin="0,0,5,0" Width="32" />
<TextBlock Text="Netpractise" FontSize="26.667" Foreground="Black" FontFamily="Moire Light" x:Name="editing" FontWeight="ExtraBold" Height="32"/>
</StackPanel>--> <Image Source="/Content/np.PNG" Grid.Row="1" Margin="100,0,0,0" HorizontalAlignment="Left" /> <Rectangle Height="5" Fill="#FFA0DA0A" Width="200" HorizontalAlignment="Left" Margin="100,0,0,0"
Grid.Row="2"/> <!--<TextBlock Text="digital communication innovation" Loaded="onTextBoxDigitalLoaded" Margin="100,0,0,0" HorizontalAlignment="Left" FontFamily="Moire" FontSize="13.333" Height="16" Grid.Row="3"/>-->
<Image Source="/Content/baseline.PNG" Margin="100,0,0,0" Width="300" HorizontalAlignment="Left" Grid.Row="3"/> <!-- Progress bar--> <Rectangle Fill="#FF24A9F3" Height="5" HorizontalAlignment="Left" x:Name="uxProgress"
Grid.Row="2"/> <!--floating text boxes--> <TextBlock x:Name="textBlock" HorizontalAlignment="Left" TextWrapping="Wrap" Text="Be it Videos, Images, Flash or Audio we support all."
VerticalAlignment="Center" Grid.Row="4" Height="400"
Width="510" Foreground="#FF24A9F3" FontSize="48" FontFamily="Segoe WP Light" RenderTransformOrigin="0.5,0.5"
Loaded="onTextBoxLoaded" Margin="20,0,0,0"> <TextBlock.RenderTransform> <TransformGroup> <TranslateTransform x:Name="myTranslate"/> </TransformGroup> </TextBlock.RenderTransform> <!--The story board can be placed
within this to run from XAML or JS functions can be used.--> <!--<TextBlock.Triggers>
<EventTrigger RoutedEvent="TextBlock.Loaded">
<BeginStoryboard>
</BeginStoryboard>
</EventTrigger>
</TextBlock.Triggers>--> </TextBlock> <TextBlock x:Name="txtProgressPercentage" Grid.Row="4" HorizontalAlignment="Right" Margin="0,-150,-170,0" VerticalAlignment="Center" TextWrapping="Wrap" Height="358" Width="651"
FontSize="300" Foreground="#FFE4E4E4"/> </Grid>
2. Code the logic in JavaScript file
When you add a new SLJS page in your application, it also creates an underlying .js file where you can write your code logic.
All I had to do was to create 3 functions in it ; they are:
onSourceDownloadProgressChanged
This is to update the progress bar and the big textblock that shows % update in numbers.
var i = 0;
function onSourceDownloadProgressChanged(sender, eventArgs)
{
sender.findName("txtProgressPercentage").Text = Math.round(Math.round((eventArgs.progress * 1000)) / 10, 1).toString();
//get browser width var width = window.document.body.clientWidth;
sender.findName("uxProgress").Width = (width * eventArgs.progress);
}
onTextBoxLoaded
This is to trigger the first story board.
function onTextBoxLoaded(sender, eventArgs)
{
// Retrieve the Storyboard and begin it. sender.findName("sb1").begin();
}
onCompleted
This is to change the Text of the floating Text box and start the Storyboard again with updated text.
function onCompleted(sender, eventArgs)
{
try{
i++;
sender.findName("myTranslate").X = 0;
switch (i) {
case 1:
sender.findName("textBlock").Text = "This is my first content";
break;
case 2:
sender.findName("textBlock").Text = "This is my second content";
break;
case 3:
sender.findName("textBlock").Text = "This is my third content.";
break;
case 4:
sender.findName("textBlock").Text = "This is my fourth content";
break;
case 5:
sender.findName("textBlock").Text = "This is my fifth content.";
break;
case 6:
sender.findName("textBlock").Text = "This is my sixth content";
i = 1;
break;
}
}catch(e){
}
sender.findName("sb1").begin();
I have 6 text block contents that show up on the screen one by one. You can have as many as you want and can also pull them from a collection if that's what you need.
3. Updating the Default.html or Default.aspx page
This is an important and the last step to hook the splash screen we created to our Silverlight application.
Just add a few parameters and a link to the JavaScript file just below the </head> tag.
<script type="text/javascript" src="splashscreen.js"></script>
The following new parameters go in <div id="silverlightControlHost">:
<param name="splashscreensource" value="SplashScreen.xaml" /> <param name="onSourceDownloadProgressChanged" value="onSourceDownloadProgressChanged" />
The first parameter just tells your app which splash screen to load at startup and the second one calls the js function that eventually updates the progress bar and % progress textblock with numbers.