The Slider control in Silverlight 5 is used to allow a user to select from a range of values by sliding it along a track. You can create a horizontal or vertical slider. You can specify the range of values and the precision of movement too. The Slider class that represents this control is defined in the System.Windows.Controls namespace. Some of the commonly used properties of this class are:
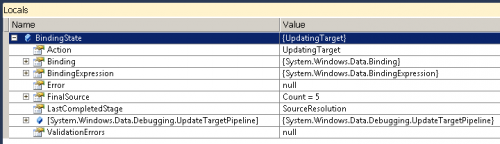
To demonstrate the use of the Slider control, let us create a TextBox with some text which is enlarged or shrunk depending upon the slider value. Create a Silverlight 5 application and replace the Grid tag with the Canvas tag. Drag and drop the Slider from the Toolbox into your XAML code (between the Canvas tags). Also drag and drop an TextBox control. The sole reason for using a TextBox here instead of TextBlock is that a TextBlock does not support the Background property and we wish to specify a background here for the control containing the text.
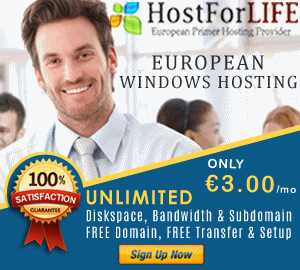
Configure the properties of the controls as shown below:
<UserControl x:Class="Imager.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="640" d:DesignHeight="480">
<Canvas x:Name="LayoutRoot" Width="640" Height="480">
<TextBox Name="txtMsg" Canvas.Left="40" Canvas.Top="30" Height="22" Width="132" Text="Silverlight Rocks!" Background="Aqua" IsReadOnly="True" />
<Slider Name="slider" Background="Transparent" Height="25" Width="150" Maximum="150" Minimum="0" SmallChange="5" ValueChanged="Slider_ValueChanged" IsDirectionReversed="False" ></Slider>
</Canvas>
</UserControl>
The code accomplishes the following:
- Creates a canvas of height 480 and width 640
- Creates a TextBox with the text "Silverlight Rocks!" and positions it on the canvas at 40,30 location.
- Creates a Slider control with transparent background, maximum 150 and minimum 0, small change (precision of movement) as 5 and height and width as 25 and 150 respectively.
- Creates an event handler for Value Changed event of Slider.
Now open the MainPage.xaml.cs and add the following code:
public partial class MainPage : UserControl
{
double start, end, height, width, size;
public MainPage()
{ InitializeComponent();
//original values of the slider
start = slider.Minimum;
end = slider.Maximum;
//original height and width of the text box
height = txtMsg.Height;
width = txtMsg.Width;
size = txtMsg.FontSize;
}
private void Slider_ValueChanged(object sender, RoutedPropertyChangedEventArgs<double> e)
{
// If slider has reached the beginning
if (e.NewValue == start)
{
txtMsg.Height = height;
txtMsg.Width = width;
txtMsg.FontSize = size;
}
//if slider has moved forward
if (e.NewValue > e.OldValue)
{
txtMsg.Height = height + e.NewValue;
txtMsg.Width = width + e.NewValue;
txtMsg.FontSize += 1;
}
else //if slider has moved backward
{
txtMsg.Height = txtMsg.Height - (e.OldValue-e.NewValue);
txtMsg.Width = txtMsg.Width - (e.OldValue - e.NewValue);
txtMsg.FontSize -= 1;
}
}
}
The logic in this code is self explanatory from the comments. When you build and execute your solution and move the slider, you will see the text increasing or decreasing in size. Though this was a simple demonstration, in actual scenarios, you can customize the Slider control through its various properties. You can also enhance its design characteristics in Expression Blend 4 if required.