Today, I will eplain about a simple way to upload file in Silverlight. Uploading files is quite an easy one in Silverlight: it’s basically just a request made to another server and the file contents are passed in this request. A possible way of implementing this is by using the WebClient class:

private void UploadFile()
{
FileStream _data; // The file stream to be read
string uploadUri;
byte[] fileContent = new byte[_data.Length]; // Read the contents of the stream into a byte array
int bytesRead = _data.Read(fileContent, 0, CHUNK_SIZE);
WebClient wc = new WebClient();
wc.OpenWriteCompleted += new OpenWriteCompletedEventHandler(wc_OpenWriteCompleted);
Uri u = new Uri(uploadUri);
wc.OpenWriteAsync(u, null, new object[] { fileContent, bytesRead }); // Upload the file to the server
}
void wc_OpenWriteCompleted(object sender, OpenWriteCompletedEventArgs e) // The upload completed
{
if (e.Error == null)
{
// Upload completed without error
}
The above solution does the job of uploading the file well. However it does not indicate file upload progress at all: when uploading large files or when having slow internet connection this behaviour would be desirable.
Silverlight has no built-in way to monitor the number of bytes sent which means that the only way to indicate upload progress is sending the file to the server in multiple, smaller chunks. Of course this behaviour needs support from the server side as well.
The idea is that multiple calls are made to the server, every call submitting the next chunk of the file. On the server these chunks are appended to the file.
Silverlight Code Snippet
public const int CHUNK_SIZE = 4096;
public const string UPLOAD_URI = "http://localhost:55087/FileUpload.ashx?filename={0}&append={1}";
private Stream _data;
private string _fileName;
private long _bytesTotal;
private long _bytesUploaded;
private void UploadFileChunk()
{
string uploadUri = ""; // Format the upload URI according to wether the it's the first chunk of the file
if (_bytesUploaded == 0)
{
uploadUri = String.Format(UPLOAD_URI,_fileName,0); // Dont't append
}
else if (_bytesUploaded < _bytesTotal)
{
uploadUri = String.Format(UPLOAD_URI, _fileName, 1); // append
}
else
{
return; // Upload finished
}
byte[] fileContent = new byte[CHUNK_SIZE];
_data.Read(fileContent, 0, CHUNK_SIZE);
WebClient wc = new WebClient();
wc.OpenWriteCompleted += new OpenWriteCompletedEventHandler(wc_OpenWriteCompleted);
Uri u = new Uri(uploadUri);
wc.OpenWriteAsync(u, null, fileContent);
_bytesUploaded += fileContent.Length;
}
void wc_OpenWriteCompleted(object sender, OpenWriteCompletedEventArgs e)
{
if (e.Error == null)
{
object[] objArr = e.UserState as object[];
byte[] fileContent = objArr[0] as byte[];
int bytesRead = Convert.ToInt32(objArr[1]);
Stream outputStream = e.Result;
outputStream.Write(fileContent, 0, bytesRead);
outputStream.Close();
if (_bytesUploaded < _bytesTotal)
{
UploadFileChunk();
}
else
{
// Upload complete
}
}
}
Since Silverlight is a client side technology the server side can be implemented in any language. In this example I’ve created .NET and PHP support for the server side.
.NET Server Side Code Snippet
public const int CHUNK_SIZE = 4096;
public const string UPLOAD_URI = "http://localhost:55087/FileUpload.ashx?filename={0}&append={1}";
private Stream _data;
private string _fileName;
private long _bytesTotal;
private long _bytesUploaded;
private void UploadFileChunk()
{
string uploadUri = ""; // Format the upload URI according to wether the it's the first chunk of the file
if (_bytesUploaded == 0)
{
uploadUri = String.Format(UPLOAD_URI,_fileName,0); // Dont't append
}
else if (_bytesUploaded < _bytesTotal)
{
uploadUri = String.Format(UPLOAD_URI, _fileName, 1); // append
}
else
{
return; // Upload finished
}
byte[] fileContent = new byte[CHUNK_SIZE];
_data.Read(fileContent, 0, CHUNK_SIZE);
WebClient wc = new WebClient();
wc.OpenWriteCompleted += new OpenWriteCompletedEventHandler(wc_OpenWriteCompleted);
Uri u = new Uri(uploadUri);
wc.OpenWriteAsync(u, null, fileContent);
_bytesUploaded += fileContent.Length;
}
void wc_OpenWriteCompleted(object sender, OpenWriteCompletedEventArgs e)
{
if (e.Error == null)
{
object[] objArr = e.UserState as object[];
byte[] fileContent = objArr[0] as byte[];
int bytesRead = Convert.ToInt32(objArr[1]);
Stream outputStream = e.Result;
outputStream.Write(fileContent, 0, bytesRead);
outputStream.Close();
if (_bytesUploaded < _bytesTotal)
{
UploadFileChunk();
}
else
{
// Upload complete
}
}
}
PHP Server Side Code Snippet
<?php
// This is the most basic of scripts with no try catches
$filename = isset($_REQUEST["filename"]) ? $_REQUEST["filename"] : "jjj";
$append = isset($_REQUEST["append"]);
try
{
if(!$append)
$file = fopen($filename,"w");
else
$file = fopen($filename,"a");
$input = file_get_contents ("php://input");
fwrite($file,$input);
fclose($file);
}
catch (Exception $e)
{
echo 'Caught exception: ', $e->getMessage(), "\n";
}
?>
Notes : Before running the project, set the UPLOAD_URI variable to point to the appropriate .asmx or .php file. The script is not suited for production environment because of the following:
Files are uploaded directly to the root directory of the web application. The files are created and constantly appended to. A more desirable approach would be to store the unfinished files in a temp folder until upload is complete and then move them to the upload folder
HostForLIFE.eu Silverlight 4 Hosting
HostForLIFE.eu revolutionized hosting with Plesk Control Panel, a Web-based interface that provides customers with 24x7 access to their server and site configuration tools. Plesk completes requests in seconds. It is included free with each hosting account. Renowned for its comprehensive functionality - beyond other hosting control panels - and ease of use, Plesk Control Panel is available only to HostForLIFE's customers. They offer a highly redundant, carrier-class architecture, designed around the needs of shared hosting customers.
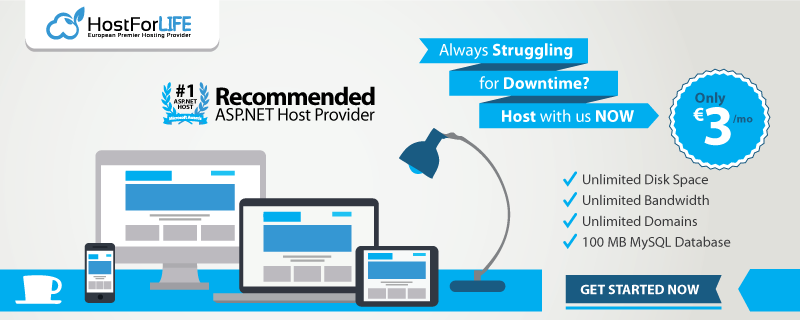