
January 6, 2015 06:57 by
Peter
In this post, I've been using Web-Service brings in Silverlight for various years now in a group of different applications with the unwieldy async BeginGetResponse, callback, EndGetResponse syntax. It's all been working extraordinary, I joyfully have a layout for this and can bash any new service integrations pretty quickly and has not been getting in the way. For a straightforward Get request they look something like this:
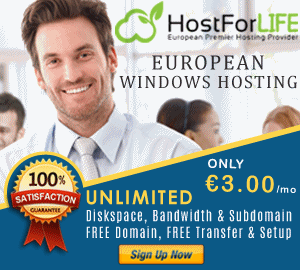
public string server = "123.123.123.123";
public void GetExample()
{
String url = "http://" + server + "/getexample";
try
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
IAsyncResult result = null;
result = request.BeginGetResponse(GetExampleCallback, request);
}
catch (Exception)
{
}
}
void GetExampleCallback(IAsyncResult ar)
{
var request = ar.AsyncState as HttpWebRequest;
var response = request.EndGetResponse(ar) as HttpWebResponse;
using (var reader = new StreamReader(response.GetResponseStream()))
{
string result = reader.ReadToEnd();
// now do something with this
}
}
Which is all great and I typically have some extra code here to callback to a representative which can then do something, for example, show the result nonconcurrently with the regular playing around of recovering this onto the GUI string using a BeginInvoke Dispatch. It then gets somewhat more muddled when you need to make a post ask for and need to push the XML parameters in an alternate asynch BeginGetRequestStream capacity which means you're getting callback after callback.
Simple enough, these can be packaged into a class for every Webservice work and can utilize some templating to diminish the exertion, yet's regardless it really dull. I've remain faithful to it in light of the fact that it meets expectations, I have an example and normally its not all that much inconvenience and once done means I can concentrate on the other fascinating bits of the application.
What I thought of resembles this:
using System.Threading.Tasks;
public string server = "123.123.123.123";
public void GetExample2()
{
String url = "http://" + server + "/getexample2";
try
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(url);
IAsyncResult result = null;
ManualResetEvent mre = new ManualResetEvent(false);
result = request.BeginGetResponse((cb) =>
{
// Callback
using (var response = request.EndGetResponse(cb) as HttpWebResponse)
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
}
}
mre.Set();
}, request);
mre.WaitOne();
}
catch (Exception)
{
}
}
Essentially this is to handle the offbeat nature. In the event that you run this in the debugger you can see the BeginGetResponse call being made. Waitone() which is the typical string stream of the operation. You can then see the debugger bounce move down to the callback. The mre.set then sets the stream to proceed in the fundamental string once the callback has completed.