New versions of the Windows Workflow Foundation (WF) and Windows Communication Foundation (WCF) are available in the .NET Framework 4.5, which is included with Windows Server 2012 and Windows 8. The upgrades improve the developer experience and the manageability of applications built using the foundations, while adding new capabilities. Some benefits, such as WF performance improvements and WCF configuration simplification can be realized for existing applications without requiring developers to make modifications. However, a majority of the enhancements are most helpful when writing new code.
WF 4.5 Brings Better Designer, Versioning
WF offers a specialized programming environment for business processes, called workflows. WF 4.5 improves on the previous version, WF 4, while providing backward compatibility.
WF Simplifies Business Process Programming
WF consists of programming interfaces, design and debugging tools, and a runtime engine (which is separate from the common language runtime included with the .NET Framework) that enable the implementation and execution of workflow processes, reducing the amount of code a developer must write.
Workflows are specialized programs that automate custom, often long-running, business processes and enforce business rules in applications. Using software to automate workflows can relieve workers from having to perform repetitive and mechanical tasks, ensure that required procedures are consistently followed, and improve the reliability, tracking, and transparency of those procedures. Several Microsoft products, including SharePoint Server, Dynamics CRM, Dynamics AX, and Team Foundation Server employ WF for workflows.
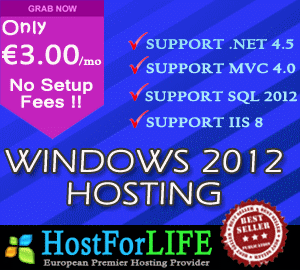
Developers build workflows in WF by assembling groups of activities, which represent the tasks and logic that comprise a business process, using the WF visual designer which generates XAML script, or by writing C# or Visual Basic (VB) code. WF includes a base set of activities that help build the basic structure of workflows, such as control loops and parallel execution paths, and developers can write custom activities that execute code specific to an application's needs. Microsoft applications integrate with WF by offering custom activities that can be used in workflows to deliver product functionality (such as checking an item into a SharePoint document library).
WF 4.5 Improves on WF 4, Maintains Compatibility
WF 4.5 builds on WF 4, which was a major upgrade that wasn't entirely backward compatible with its predecessor, WF 3.5. Microsoft calls WF 4.5 an additive update, implying that it is fully backward compatible with WF 4. WF 4.5 and WF 4 cannot coexist in a Windows installation, since installing .NET 4.5 replaces .NET 4.
Enhancements in WF 4.5 continue on themes addressed in WF 4, such as simplified development and better performance, and include the following:
Contract-first development. WF is often used in combination with WCF to implement workflows that communicate with remote Web services. Contract-first development in WF automatically generates activity objects for existing WCF services, and developers can use these in the WF designer, reducing the work required to create workflows that use Web services.
C# expressions. Code expressions can be included within workflow activities for tasks such as creating output strings. Previously, only VB could be used to write these expressions (even within C# workflow projects), but now C# is supported as well.
More state machine support. A state machine defines a process that exists within one of multiple possible states until an event causes it to move to a different state. States can be revisited indefinitely, or the process can reach a final state. State machines can describe many types of processes, such as order fulfillment for businesses or chemical reactions for scientific research. WF 4.5 adds features for building state machines, including new activities, designer tools that reduce manual steps, and debugging capabilities.
Designer improvements. A graphical designer for building WF workflows is available in Visual Studio (VS), allowing developers to visually create workflows rather than defining them entirely within code (which is also supported). The WF 4.5 designer adds search features (although not replace), an outline view (similar to Word's Navigation pane), and a pan mode, which will help developers work with large workflows. The newest designer also allows annotations to be added to activities to assist search and enable better commenting of intended functionality. Auto-connect simplifies attaching new activities into a workflow, and auto-insert helps when placing an activity between two existing activities in a workflow.
The designer can also be hosted within other applications so that users don't need to run VS to work with workflows targeted for specific solution spaces, and so that users are limited to workflow elements that are relevant to an application domain. However, not all features available in the designer in VS are available when it is hosted elsewhere (for example, C# expressions and some search capabilities are not available).
Workflow versioning. Workflow processes are prone to revisions, but WF has not previously offered versioning support, leaving the task to developers and IT personnel. WF 4.5 introduces workflow identities, which allow the assignment of names and version numbers to workflow definitions. Multiple definitions can be deployed side by side, and running instances of a workflow can be identified by the definition they are using. This allows new workflow definitions to be deployed and used by new instances while old instances run to completion using an older definition. Further, a dynamic update feature allows existing instances to convert to a later workflow definition, provided the developer defines how the conversion should be handled.
Partial trust hosting. Previously, workflow code would have access to all permissions available to the host application, but in WF 4.5, workflows can run in a partially trusted AppDomain, limiting their capabilities to work with the system. Partial trust can reduce the security risk of using workflows obtained from third parties and can make using workflows safer in hosted multitenant deployments.
Better performance. VB expression support has been modified to deliver better runtime performance, which will improve the efficiency of workflows implemented with such expressions by upgrading to WF 4.5 without rewriting code.
WCF 4.5 Eases Configuration, Adds Communications Options
WCF provides developers with a message-oriented API that can be used to communicate over local networks or across the Internet using protocols such as HTTP, TCP, and Microsoft Message Queuing (MSMQ), reducing the amount of code and time required to integrate applications and enabling the implementation of service-oriented architectures.
The power of WCF is its ability to decouple a Web service's internal logic from the protocols used to send and receive messages, allowing either to be modified without affecting the other. The Web service logic is contained in the source code, while the communications protocol information is contained in a separate configuration file. This allows developers to create business logic and IT administrators to determine the best way to access that logic. The model also allows Microsoft to focus development on a single infrastructure and tool set that handles multiple messaging protocols, rather than developing separate solutions for each protocol.
WCF 4.5 aims to reduce configuration complexity and developer effort while improving performance and adding new communications options with enhancements that include the following:
Configuration file simplification. WCF uses XML-based configuration files to separate connection protocol information from code, but these files can be tedious to edit. Properties set to default values can be excluded in WCF 4.5 configuration files, shortening the files and reducing the complexity of working with them. Also, the VS XML editor now provides tooltips when working with a WCF configuration file to help developers understand elements and properties without opening separate documentation.
Asynchronous methods. .NET 4.5 has new elements that simplify the development effort required to write applications that perform tasks in parallel. Methods can be called asynchronously (so they run in a separate thread while the calling code path continues) with much less coding overhead than previously. WCF 4.5 supports methods that take advantage of the new .NET 4.5 asynchronous capabilities, helping developers create better performing WCF applications.
Portable libraries. A portable .NET Framework class library can run on different platforms such as Windows 8, Windows Phone 8, and the Windows Store, eliminating the need to write multiple versions of code for tasks that run the same way on each platform. WCF 4.5 code (with some exceptions) can be used in portable class libraries, so an application's WCF code doesn't have to be rewritten for each platform.
Better message streaming. WCF 4.5 offers improved performance when messages are streamed by using asynchronous streaming, which allows process threads to be closed and reused while waiting on a response (previously, threads would hold system resources while waiting for a response). Also, performance is improved when messages are streamed by a service hosted in IIS, since ASP.NET will now send the message to WCF before the entire message is received (previously, ASP.NET would wait until the full message was received and buffered). This reduces memory usage and enables WCF to respond more quickly.
UDP and WebSocket bindings. WCF uses bindings to define how services communicate. Several binding types, such as HTTP, TCP, and MSMQ are included with WCF, and developers can define custom binding types for other communication mechanisms. WCF 4.5 adds bindings for UDP (which supports message broadcasting to multiple clients without soliciting a response) and WebSocket (which provides two-way TCP communication using HTTP or HTTPS ports with reduced overhead and better performance than HTTP).