Introduction
Silverlight is evolving with a lot of new features in each and every version release. The AutoComplete text feature is one such example. In this article I will demonstrate the implementation of the AutoComplete text feature in a Silverlight application. I will also create a sample Silverlight application to help explain the code. I have used Silverlight 4.0 and Visual Studio 2010 for developing the sample application.
AutoComplete Functionality
AutoComplete text functionality is not only a fancy effect but it's also a pretty useful feature from a user prospective and this feature is available in most of the latest applications. As the user enters text in a text box, a list of values gets populated and are listed in a similar fashion to that of a drop down based on the entered text. So the user is able to see the possible suggestions and can select a value from them or they also have the freedom to enter their own text as the base control is a textbox.
Some popular websites implementing the auto complete functionality are www.google.com,www.youtube.com, etc.,
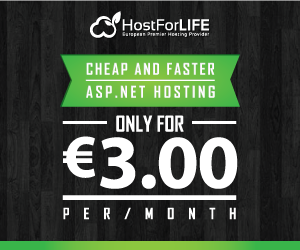
Silverlight AutoCompleteBox Control
Implementing the autocomplete functionality in a Silverlight application is pretty straight forward because of the availability of the AutoCompleteBox control. This control is available in Silverlight 3.0 and higher versions. The developer only needs to set the ItemSource property of the AutoCompleteBox control with the value collection that is to be listed. The rest will be taken care by the control itself.
Below are some of the useful settings that can be leveraged from the AutoCompleteBox control.
- FilterMode – Specifies the filter mode to display the data (StartsWith, Contains, Equals, etc.,)
- MinimumPrefixLength – Minimum prefix length for the auto complete feature to be triggered
- MaxDropDownHeight – Maximum height of the dropdown
- IsTextCompletionEnabled – If set to true then the first match found during the filtering process will be populated in the TextBox
Silverlight AutoCompleteBox Implementation
In this section we will create a sample Silverlight window implementing the autocomplete text feature. In the MainWindow.xaml add an AutoCompleteBox control and set the basic properties. Below is the code:
<UserControl xmlns:sdk="http://schemas.microsoft.com/winfx/2006/xaml/presentation/sdk"
x:Class="AutoCompleteBoxSample.MainPage"
xmlns=http://schemas.microsoft.com/winfx/2006/xaml/presentation
xmlns:x=http://schemas.microsoft.com/winfx/2006/xaml
xmlns:d=http://schemas.microsoft.com/expression/blend/2008
xmlns:mc=http://schemas.openxmlformats.org/markup-compatibility/2006
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<Canvas>
<sdk:Label Content="Enter the city: " Margin="46,76,264,198" />
<sdk:AutoCompleteBox Height="28" HorizontalAlignment="Left" Margin="142,77,0,0" FilterMode="StartsWith"
MinimumPrefixLength="1" MaxDropDownHeight="80" Name="autoCompleteBox1" VerticalAlignment="Top" Width="120"
Canvas.Left="-6" Canvas.Top="-5" />
</Canvas>
</Grid>
</UserControl>
namespace AutoCompleteBoxSample
{
public partial class MainPage : UserControl
{
List<string> _cities;
public MainPage()
{
InitializeComponent();
autoCompleteBox1.ItemsSource = PopulateCities();
}
private IEnumerable PopulateCities()
{
_cities = new List<string>();
_cities.Add("Boston");
_cities.Add("Bangalore");
_cities.Add("Birmingham");
_cities.Add("Auckland");
_cities.Add("Amsterdam");
_cities.Add("Aspen");
return _cities;
}
}
}
Run the application and you will see the figure below:
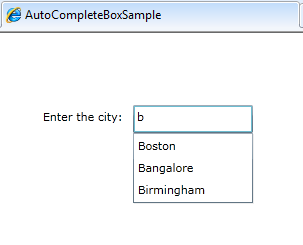
Using a DomainDataSource
In the above case we had the data directly in the application and it was hence hard-coded. In case if the data lies in the database, then the WCF RIA service and the DomainDataSource comes into play. Create a WCF RIA service and hook up the service to expose the data in the table through a generated data context method. Use a DomainDataSource to act as an ItemSource for the AutoCompleteBox control.
Below is the XAML code:
<Canvas>
<riaControls:DomainDataSource AutoLoad="True"
QueryName="GetCities"
x:Name="CityDataSource">
<riaControls:DomainDataSource.DomainContext>
<web:MyDatabaseContext />
</riaControls:DomainDataSource.DomainContext>
</riaControls:DomainDataSource>
<sdk:Label Content="Enter the city: " Margin="46,76,264,198" />
<sdk:AutoCompleteBox Height="28" ItemsSource="{Binding Data, ElementName=CityDataSource}"
HorizontalAlignment="Left" Margin="142,77,0,0" FilterMode="StartsWith" MinimumPrefixLength="1" MaxDropDownHeight="80"
Name="autoCompleteBox1" VerticalAlignment="Top" Width="120" Canvas.Left="-6" Canvas.Top="-5" />
</Canvas>