When you worked with Silverlight to create an GUI to let the user to upload the file, it is important to create a progress bar to make the user aware of the uploading progress.Today, I will show you how to create a progress bar while uploading a file.
Here is the sample of the progress bar that I've created:
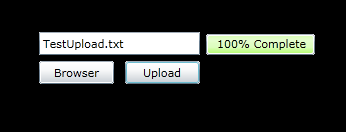
Several resources can be obtained online, but the explaination is either not clear enough, or there are redundant codes confusing people. Here, the code is as simplest as possible, with the assumption that,
a) there is no connection lost between the client and the server during the process
b) there is no data corrupted during the transmission
c) small file size (can be edited)
Before we go with code, first you can observe that there are 4 UI components:
a) Browser button
b) Textbox shows file name (can be disabled)
c) Upload button
d) Progress bar + labels
Browser Button
Here's the code to create the browser button:
OpenFileDialog dialog = new OpenFileDialog(); //OpenFileDialog will open a file dialog which allows the use to browser the wanted file.
if ((bool)dialog.ShowDialog())
{
globalFileStream = dialog.File.OpenRead();
....
....
}
Upload Button
An always updating progress bar means that, the upload progress has to be done 'Chunk' by 'Chunk'. To achieve this, we read the file stream 'Chunk' by 'Chunk and upload it.
Step 1 : Send First Chunck
int steps = (int)(fileLength / (long)CHUNK_SIZE);
progressBar1.Minimum = 0; //set prograssbar info
progressBar1.Maximum = steps;
int read = 0;
byte[] buffer = null; //buffer to store the file stream chunk by chunk
if (globalFileStream.Length <= CHUNK_SIZE) //consider if the file size is smaller than the predefined chunk size
{
buffer = new byte[(int)globalFileStream.Length];
}
else
{
buffer = new byte[CHUNK_SIZE];
}
read = globalFileStream.Read(buffer, 0, buffer.Length);
filePosition += read;
myUpload.BeginUploadAsync(fileName, destinationFolder, buffer); //begin upload the first chunk
Step 2: Sends second and subsequent chunk
UpdateProgressBar(); //Update progress bar
if (filePosition < fileLength)
{
int read = 0;
int readSize = CHUNK_SIZE;
byte[] buffer = null;
long diff = fileLength - filePosition;
if (diff < CHUNK_SIZE)
{
readSize = (int)diff;
}
buffer = new byte[readSize];
globalFileStream.Seek(filePosition, SeekOrigin.Begin);
read = globalFileStream.Read(buffer, 0, readSize);
filePosition += read;
myUpload.ContinueUploadAsync(theFileName, "here", buffer);
For your information, progress bar provided by Silverlight doesn't have the percentage displayed. Hence, I additionally put a label on top of the progress bar. The label contents are the percentage of the file stream sent to the server.
Silverlight 6 with Free ASP.NET Hosting
Try our Silverlight 6 with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc. You will not be charged a cent for trying our service for the next 3 days. Once your trial period is complete, you decide whether you'd like to continue.

